Now, with the new Beta version of the Gemini Dart SDK plug-in, developers can seamlessly integrate AI capabilities into their Flutter applications.
Let’s dive into the power and best practices of Gemini AI to introduce new functionalities to create much richer digital experiences.
Having access to Google's generative AI SDK allows new possibilities in app development, as well as enhancing existing functionalities. We will showcase how to integrate Gemini AI into a Flutter app.
Getting an API key
Before diving into the code, we have to get an API key from Google AI Studio. If you are having issues trying to access Google AI Studio with a work account, you can either contact your administrator to enable Early Access apps or use a personal Gmail account, since those have the access enabled already.
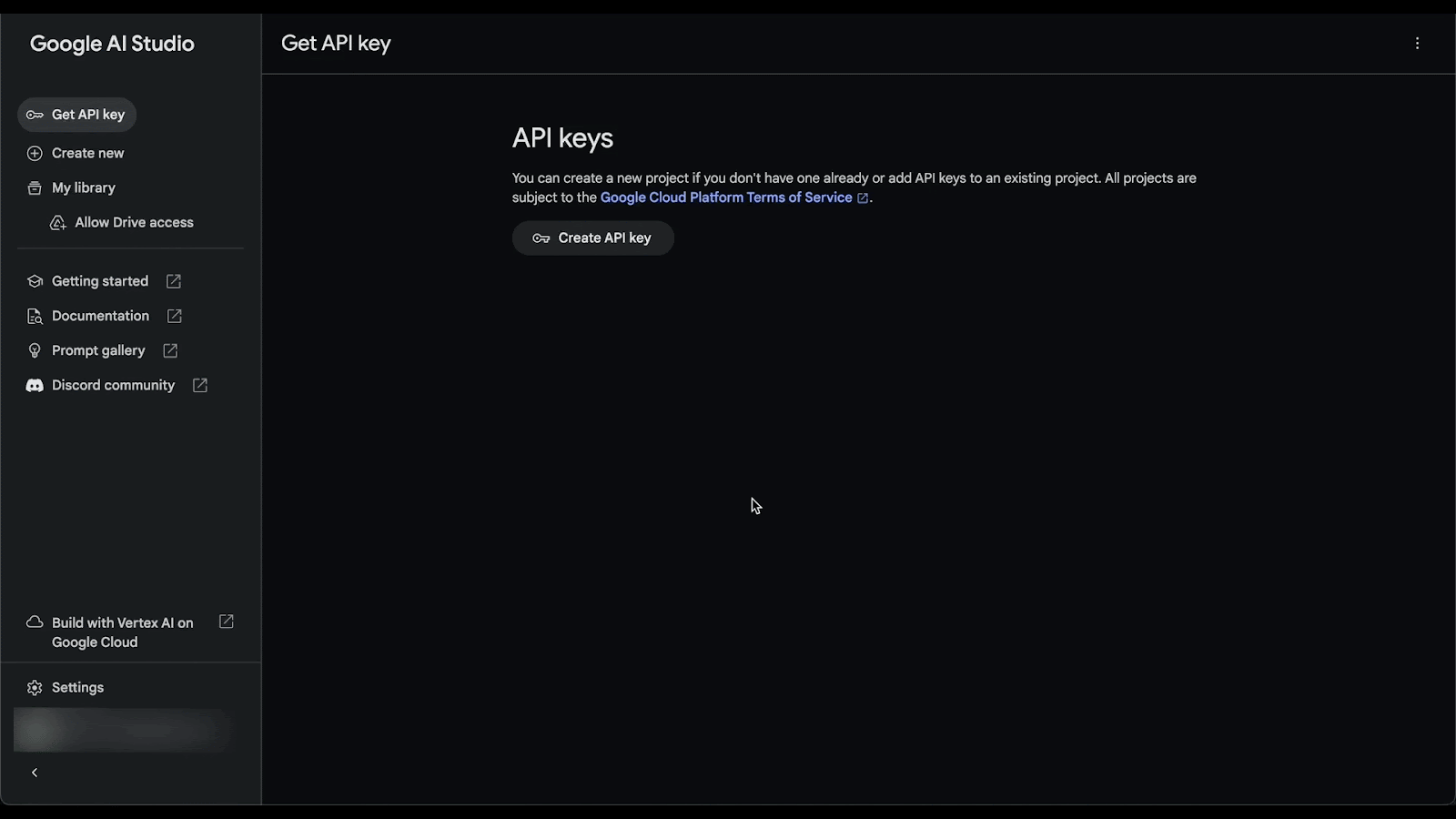
Adding the SDK to an app
We will be using the Google Generative AI SDK for Dart, which is already available in pub.dev.
To use the package in your app, add the dependency to your pubspec.yaml file.
Remember to check the package's latest instructions on how to add it to your project if you are having issues.
Best practices
Now that we have the package available in the app, let's see how to use it effectively.
Creating a Gemini client
At VGV we believe that a layered architecture is the best way to build maintainable, testable, performant, and scalable code. Following this pattern, we will create a GeminiClient that will be responsible for interacting with the Generative AI SDK and parsing the responses as needed.
In this example, we're just showing how to generate content from a text prompt, but we encourage you to play with other types of content such as images with the Content.multi() constructor, or even starting a chat session with Gemini with the _model.startChat() method!
Provide the API key securely
The API key accesses the model on your behalf, you should keep it safe and private. One way of doing so is by providing it as an environment variable when running our app. First, we will build the logic in our app to retrieve it.
Then, when running your app, provide the key.
Organize and add context to prompts
There are plenty of use cases for generative AI to be used in an app, but don’t miss the opportunity to combine user prompts with additional context.
Let’s say that you have a story-teller feature in your app powered by Gemini. The user can prompt a summary of the plot or some events that the story should have, but you, as the developer, can add some context to enhance the story and add an overarching theme before sending the request to Gemini. This and other techniques are explained more deeply in the Gemini API documentation.
Let’s see it in action
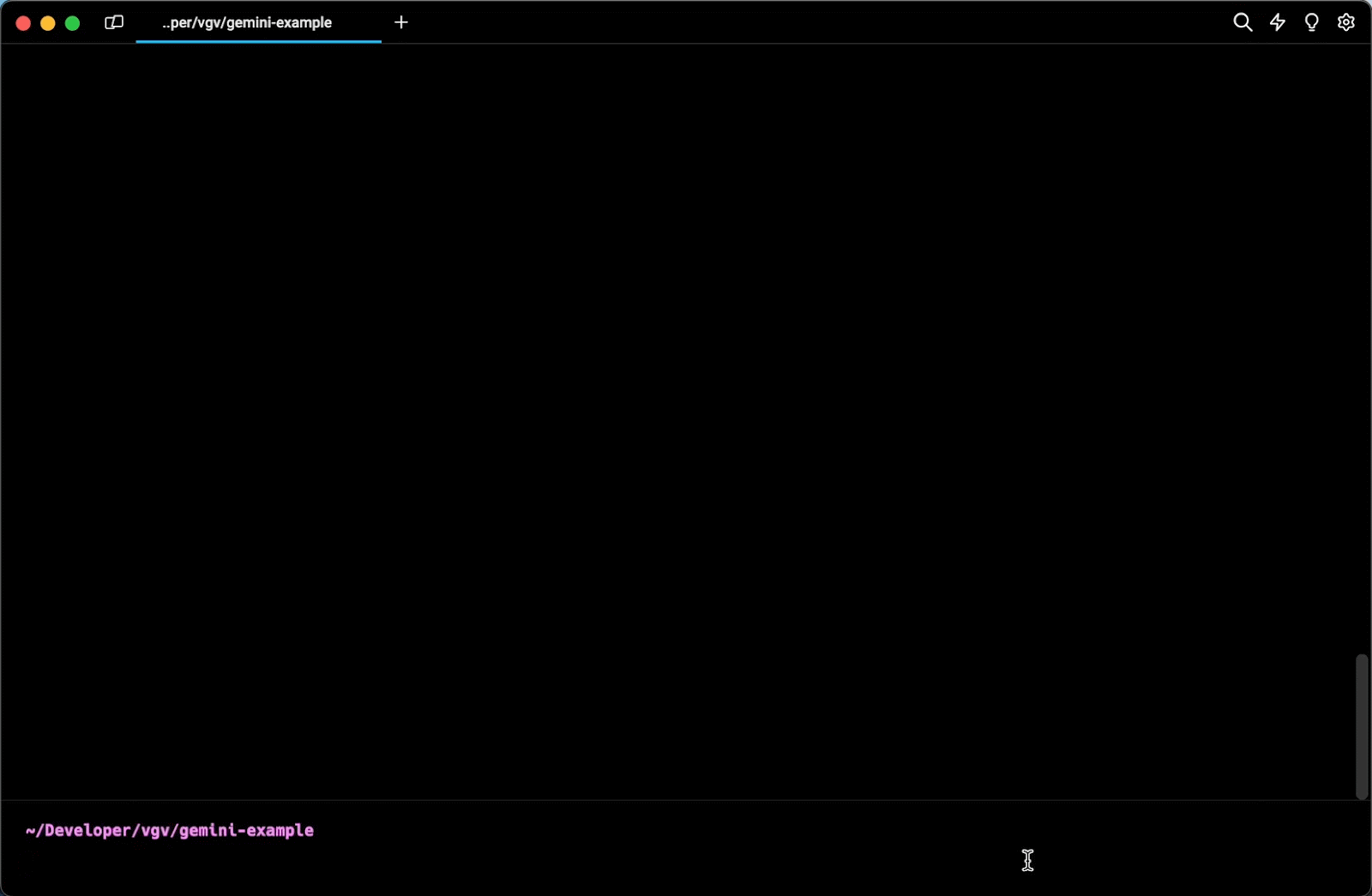